The number 3.141592653589793 rounded to 2 decimal places is 3.14.
You can use the `round` function in Python to achieve this. If you have any other numbers you'd like to round or any other questions about Python, feel free to ask!
Python中保留小数的实用方法与技巧
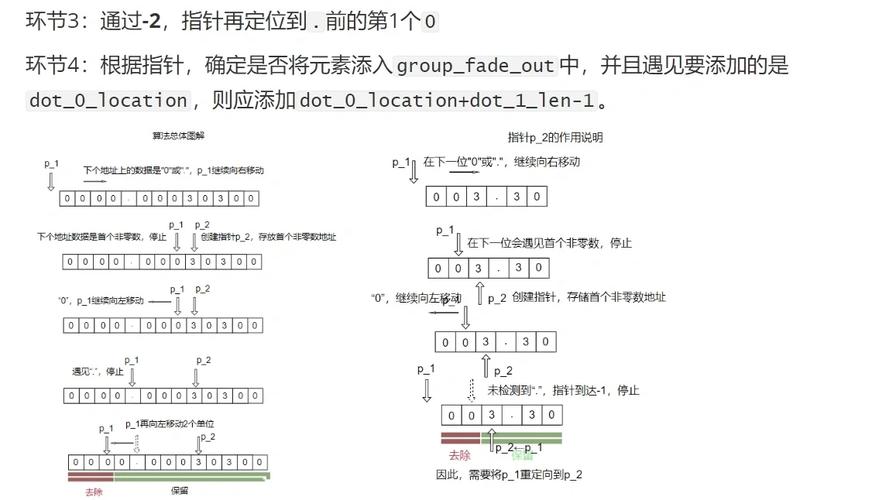
在Python编程中,保留小数是一个常见的操作,尤其是在处理金融数据、科学计算和日常应用时。本文将详细介绍Python中保留小数的几种常用方法,帮助您在编程中更加高效地处理小数数据。
一、使用内置函数round()保留小数
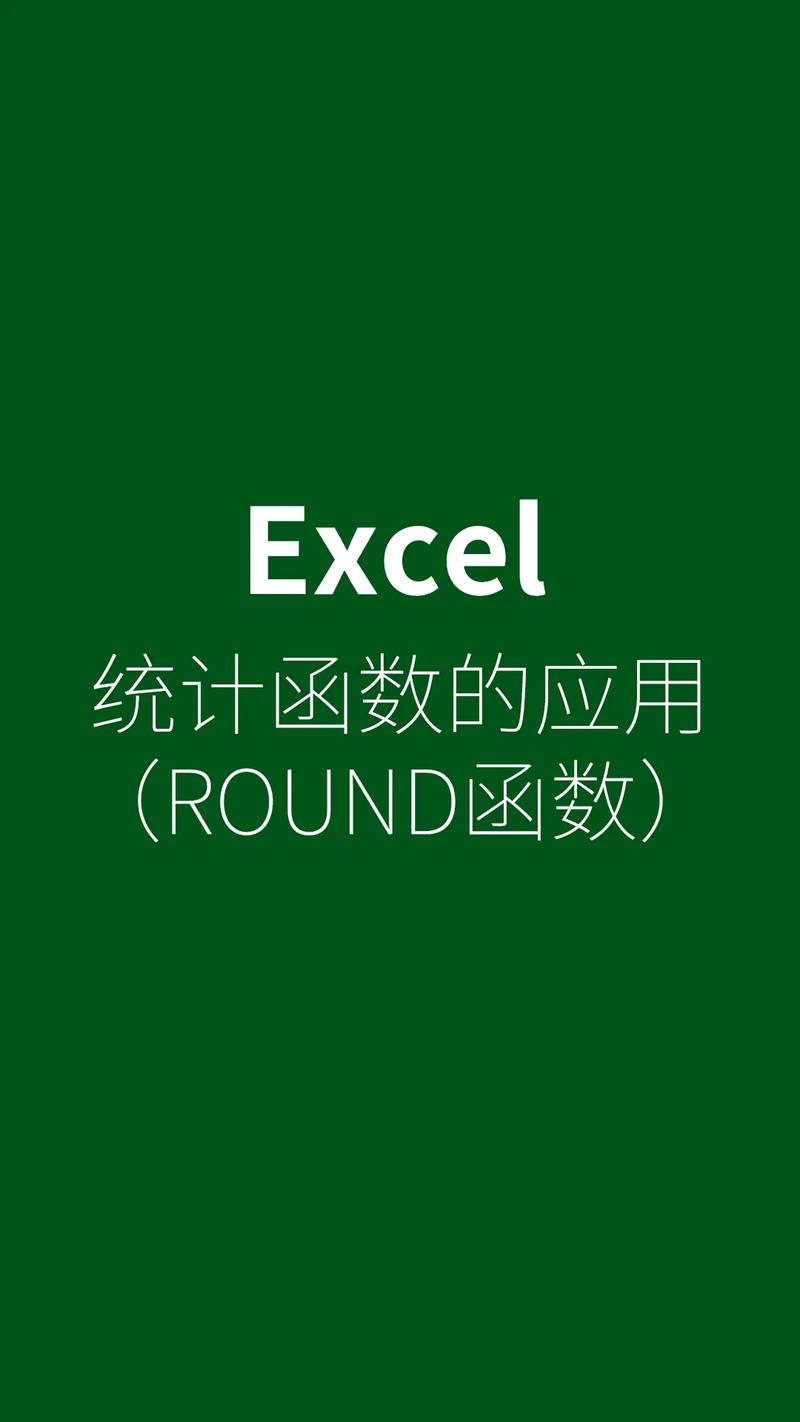
Python的内置函数`round()`可以用来对浮点数进行四舍五入,保留指定的小数位数。其基本语法如下:
round(number, ndigits)
其中,`number`是需要四舍五入的数字,`ndigits`是保留的小数位数。如果`ndigits`为负数,则表示保留整数部分的位数。
例如:
print(round(3.14159, 2)) 输出: 3.14
print(round(3.14159, -1)) 输出: 3
二、使用字符串格式化保留小数
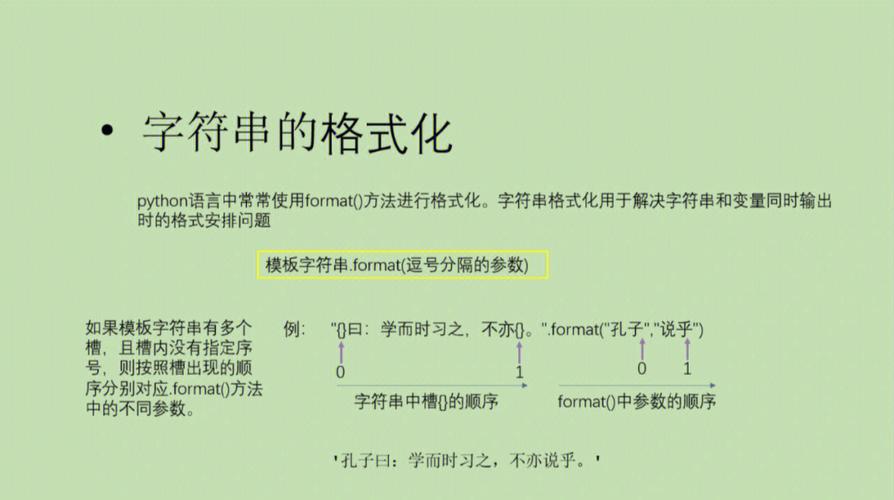
Python提供了多种字符串格式化方法来保留小数,其中最常用的是`format()`函数和f-string(格式化字符串字面量)。
1. 使用format()函数
格式化字符串的`format()`函数可以用来保留小数,其语法如下:
format(value, '.{}f').format(number)
其中,`number`是需要格式化的数字,`{}`是一个占位符,用于指定小数位数。
例如:
print(format(3.14159, '.2f')) 输出: 3.14
print(format(3.14159, '.3f')) 输出: 3.142
2. 使用f-string
Python 3.6及以上版本引入了f-string,它提供了一种更简洁的字符串格式化方法。
例如:
number = 3.14159
print(f'{number:.2f}') 输出: 3.14
print(f'{number:.3f}') 输出: 3.142
三、使用decimal模块精确保留小数
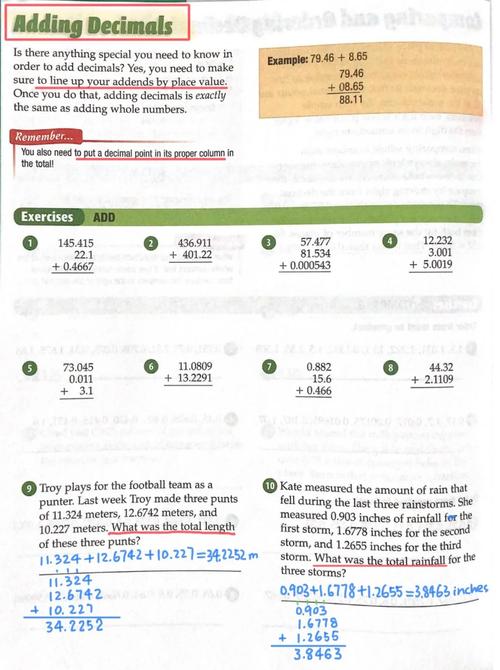
当需要高精度的小数运算时,可以使用Python的`decimal`模块。该模块提供了一个`Decimal`数据类型,可以用来进行精确的小数运算。
首先,需要导入`decimal`模块,然后创建`Decimal`对象,并使用`quantize()`方法来指定小数位数。
例如:
from decimal import Decimal, ROUND_HALF_UP
number = Decimal('3.14159')
formatted_number = number.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
print(formatted_number) 输出: 3.14
在Python中,保留小数的方法有很多,可以根据实际需求选择合适的方法。`round()`函数适用于简单的四舍五入操作,字符串格式化方法适用于快速格式化输出,而`decimal`模块则适用于需要高精度小数运算的场景。
掌握这些方法,可以帮助您在Python编程中更加灵活地处理小数数据。