Python实用小程序:轻松提升工作效率与生活趣味
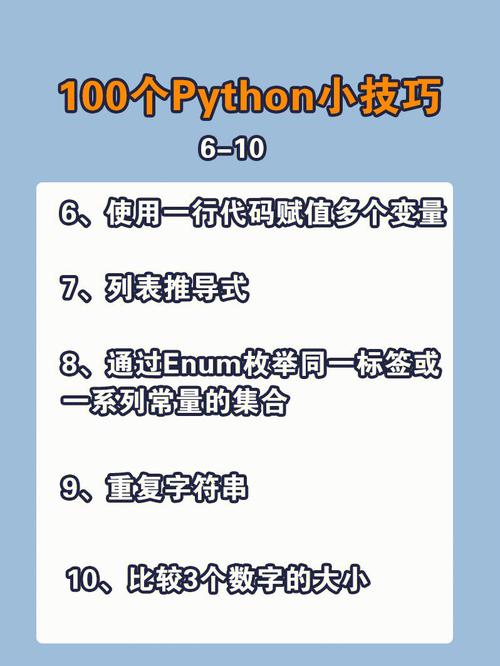
Python作为一种功能强大的编程语言,因其简洁的语法和丰富的库支持,在各个领域都得到了广泛的应用。本文将介绍一些实用的Python小程序,帮助您提升工作效率,同时也能为您的日常生活增添趣味。
一、Python编程环境搭建
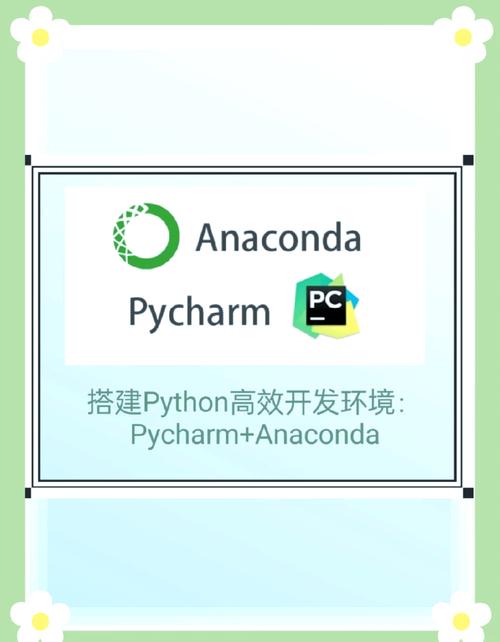
PyCharm:一款功能强大的Python集成开发环境(IDE),支持代码调试、版本控制等功能。
VS Code:一款轻量级的代码编辑器,通过安装Python插件,可以提供Python开发所需的特性。
Sublime Text:一款简洁的代码编辑器,通过安装Python插件,可以支持Python开发。
二、Python实用小程序案例
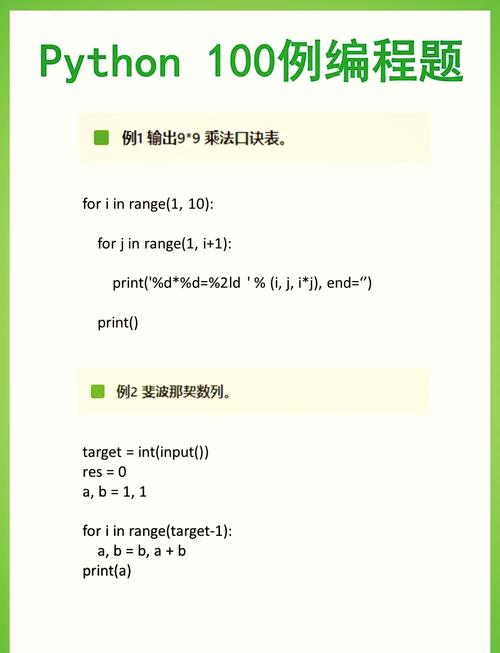
1. 文件夹清理工具
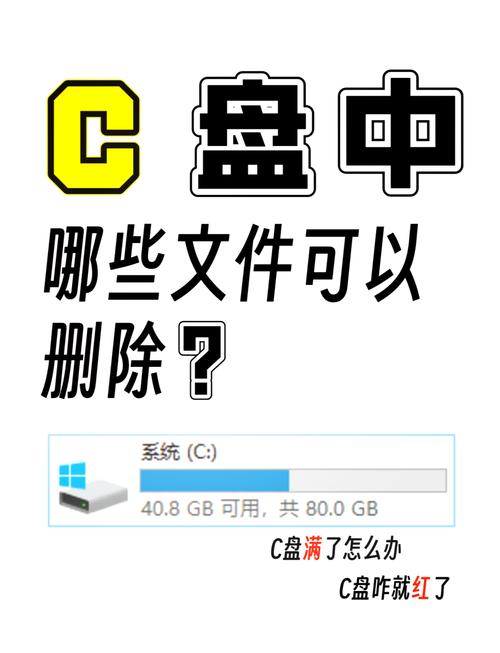
这个小程序可以帮助您清理电脑中的临时文件和缓存,释放磁盘空间。
import os
import shutil
def clean_folder(folder_path):
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith('.tmp') or file.endswith('.log'):
os.remove(os.path.join(root, file))
if __name__ == '__main__':
folder_path = input('请输入要清理的文件夹路径:')
clean_folder(folder_path)
2. 随机密码生成器
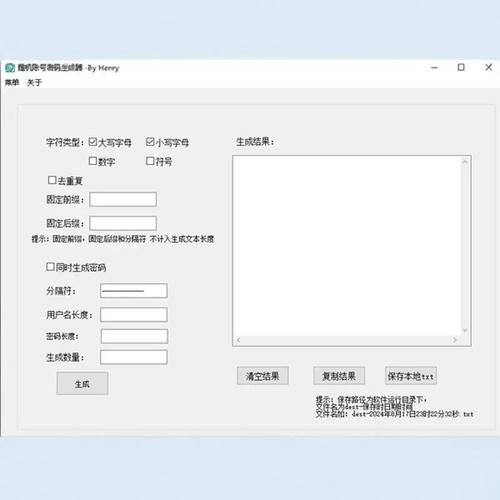
这个小程序可以帮助您生成随机密码,提高账户安全性。
import random
import string
def generate_password(length):
characters = string.ascii_letters string.digits string.punctuation
return ''.join(random.choice(characters) for i in range(length))
if __name__ == '__main__':
password_length = int(input('请输入密码长度:'))
password = generate_password(password_length)
print('生成的密码为:', password)
3. 倒计时器
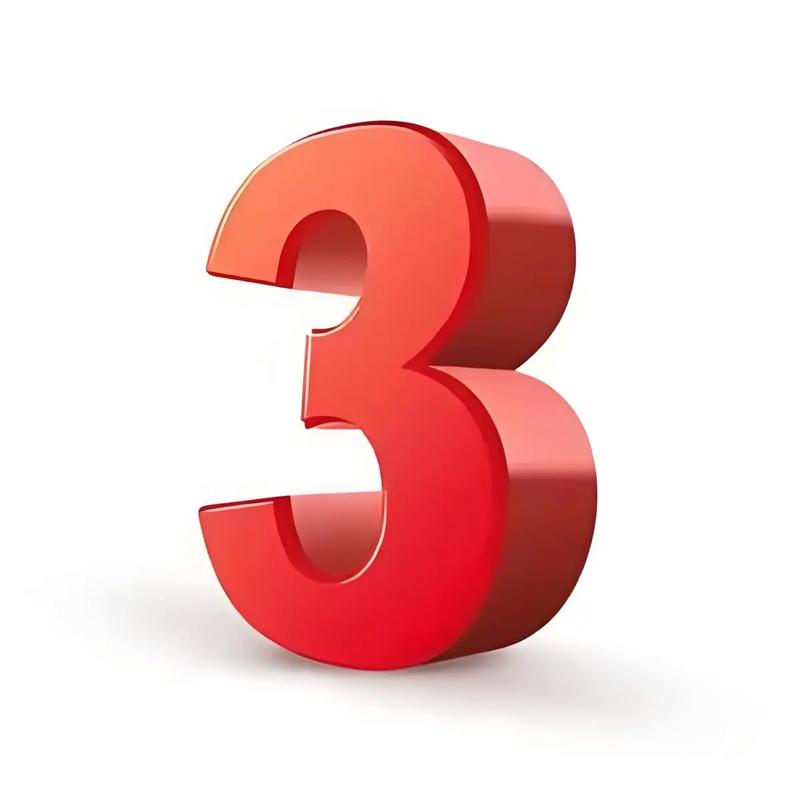
这个小程序可以作为一个简单的倒计时器,帮助您在特定时间内完成任务。
import time
def countdown(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timeformat = '{:02d}:{:02d}'.format(mins, secs)
print(timeformat, end='\\r')
time.sleep(1)
seconds -= 1
print('时间到!')
if __name__ == '__main__':
countdown(60)
4. 随机句子生成器
这个小程序可以根据用户输入的词汇,生成随机且有趣的句子。
import random
def generate_sentence(nouns, verbs, adjectives):
sentence = ''
for i in range(nouns):
sentence = random.choice(nouns) ' '
for i in range(verbs):
sentence = random.choice(verbs) ' '
for i in range(adjectives):
sentence = random.choice(adjectives) ' '
return sentence.strip()
if __name__ == '__main__':
nouns = ['苹果', '书', '电脑']
verbs = ['吃', '看', '玩']
adjectives = ['好吃的', '有趣的', '强大的']
sentence = generate_sentence(nouns, verbs, adjectives)
print('生成的句子为:', sentence)