在Vue中,你可以使用JavaScript的`Date`对象来获取当前时间。以下是一个简单的示例,展示了如何在Vue组件中获取并显示当前时间:
```javascript 当前时间: {{ currentTime }}
export default { data { return { currentTime: new Date.toLocaleString, }; }, mounted { this.updateTime; }, methods: { updateTime { setInterval => { this.currentTime = new Date.toLocaleString; }, 1000qwe2; }, },};```
在这个示例中,`currentTime` 数据属性用于存储当前时间。在组件的`mounted`生命周期钩子中,我们调用`updateTime`方法,该方法使用`setInterval`函数每秒更新一次时间。这样,页面上显示的时间就会每秒更新一次。
Vue 获取当前时间的详细指南
在开发Vue应用时,获取当前时间是一个常见的需求。这不仅用于显示时间信息,还可能用于计算时间差、设置定时器等。本文将详细介绍如何在Vue中获取当前时间,并提供一些实用的示例。
一、使用JavaScript内置函数获取当前时间
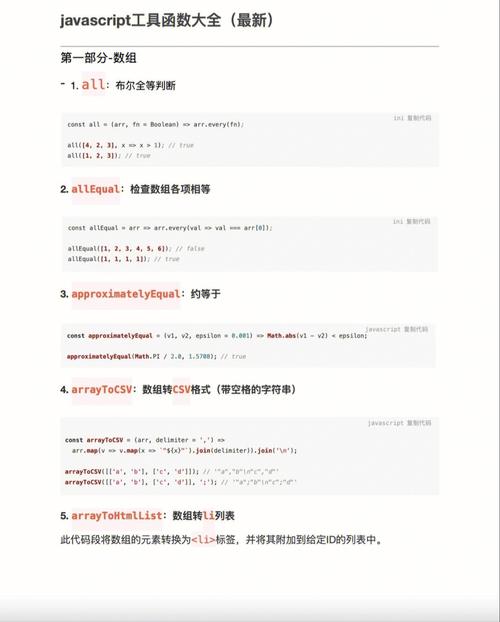
JavaScript提供了`Date`对象,可以用来获取和操作日期和时间。在Vue组件中,你可以直接使用`Date`对象来获取当前时间。
```javascript
当前时间:{{ currentTime }}
export default {
data() {
return {
currentTime: ''
};
},
mounted() {
this.currentTime = new Date().toLocaleString();
在上面的示例中,我们在`mounted`生命周期钩子中获取当前时间,并将其赋值给`currentTime`数据属性。
二、格式化时间显示
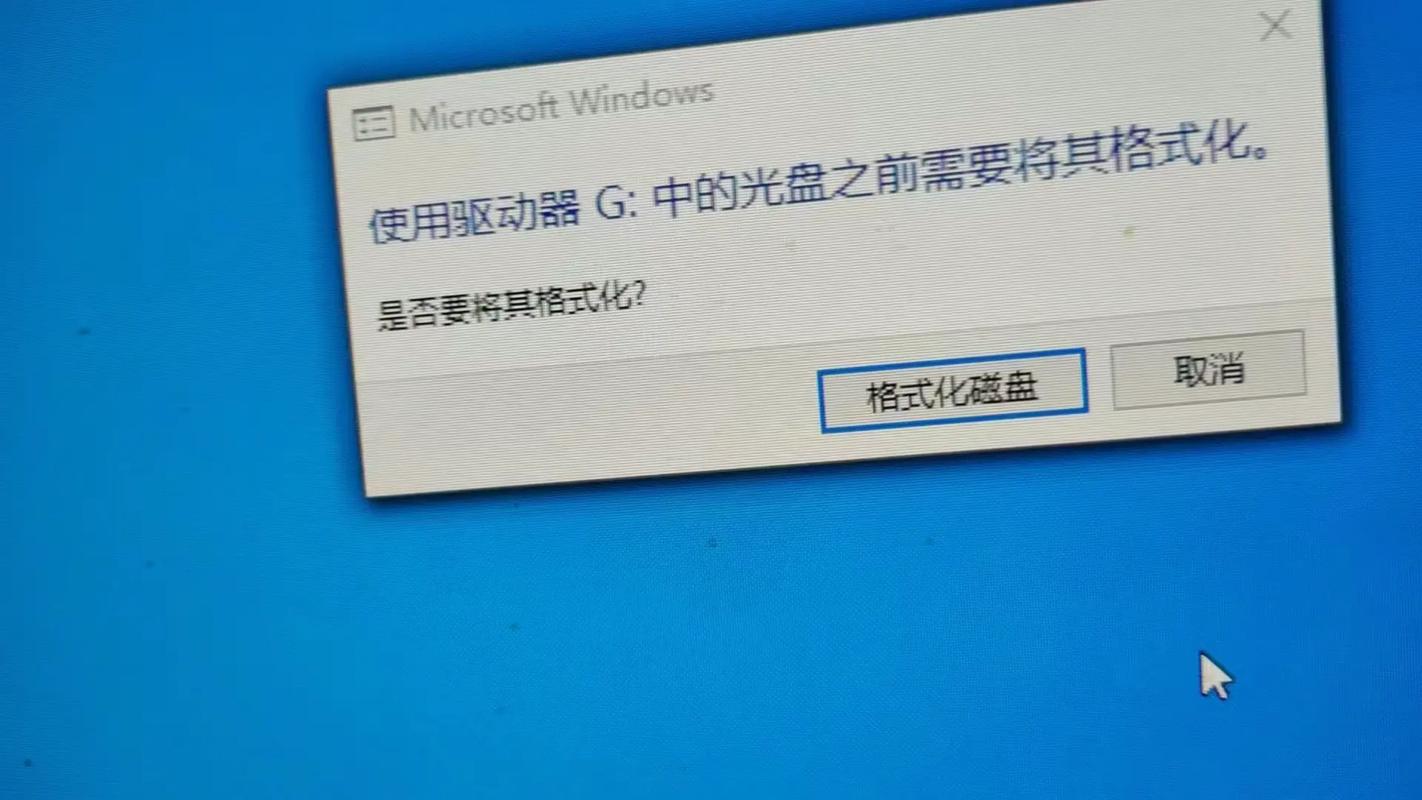
获取到当前时间后,你可能需要将其格式化为更易读的格式。Vue提供了`toLocaleString`方法,可以用来格式化时间。
```javascript
当前时间:{{ formattedTime }}
export default {
data() {
return {
formattedTime: ''
};
},
mounted() {
const now = new Date();
this.formattedTime = now.toLocaleString('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric',
hour: 'numeric',
minute: 'numeric',
second: 'numeric',
hour12: false
});
在这个例子中,我们使用`toLocaleString`方法将时间格式化为美国英语格式,包括年、月、日、小时、分钟和秒。
三、使用第三方库进行时间格式化
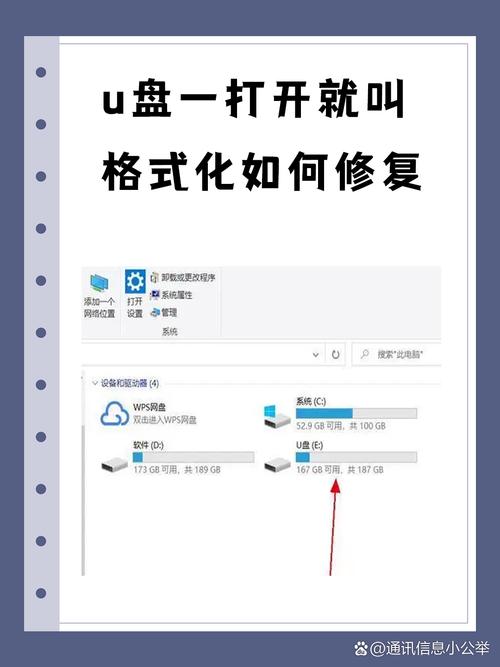
虽然`toLocaleString`方法可以满足基本的格式化需求,但对于更复杂的格式化,你可能需要使用第三方库,如`moment.js`或`date-fns`。
由于你要求不使用外部包,这里我们以`date-fns`为例进行说明。首先,你需要安装`date-fns`:
```bash
npm install date-fns
在Vue组件中使用`format`函数来格式化时间:
```javascript
当前时间:{{ formattedTime }}
import { format } from 'date-fns';
export default {
data() {
return {
formattedTime: ''
};
},
mounted() {
const now = new Date();
this.formattedTime = format(now, 'yyyy-MM-dd HH:mm:ss');
在这个例子中,我们使用`date-fns`的`format`函数将时间格式化为`年-月-日 时:分:秒`格式。
四、动态更新时间显示
在实际应用中,你可能需要动态更新时间显示,例如每秒更新一次。这可以通过设置一个定时器来实现。
```javascript
当前时间:{{ formattedTime }}
import { format } from 'date-fns';
export default {
data() {
return {
formattedTime: '',
timer: null
};
},
mounted() {
this.updateTime();
},
beforeDestroy() {
if (this.timer) {
clearInterval(this.timer);
}
},
methods: {
updateTime() {
const now = new Date();
this.formattedTime = format(now, 'yyyy-MM-dd HH:mm:ss');
this.timer = setInterval(this.updateTime, 1000);
}
在这个例子中,我们在`mounted`钩子中调用`updateTime`方法来初始化时间显示,并在`beforeDestroy`钩子中清除定时器,以避免内存泄漏。
在Vue中获取和显示当前时间是一个简单但实用的功能。通过使用JavaScript内置的`Date`对象或第三方库,你可以轻松地获取和格式化时间。同时,通过设置定时器,你可以实现动态更新时间显示。希望本文能帮助你更好地在Vue项目中处理时间相关的需求。