好的,我将为您介绍C语言中的队列。队列是一种先进先出(FIFO)的数据结构,它允许在队列的尾部添加元素,并在队列的头部移除元素。
以下是C语言中实现队列的步骤:
1. 定义队列的结构体:队列通常由一个数组或链表组成,并包含队列头和队列尾的指针。
2. 初始化队列:在队列开始使用之前,需要初始化队列头和队列尾的指针。
3. 入队操作:将一个元素添加到队列的尾部。
4. 出队操作:从队列的头部移除一个元素。
5. 检查队列是否为空:在执行出队操作之前,需要检查队列是否为空。
6. 获取队列的长度:返回队列中元素的数量。
7. 清空队列:将队列中的所有元素移除。
8. 销毁队列:释放队列占用的内存。
以下是一个简单的C语言队列实现示例:
```cinclude include
define MAX_SIZE 100
typedef struct { int data; int front; int rear;} Queue;
void initializeQueue { q>front = 1; q>rear = 1;}
int isEmpty { return q>front == 1;}
int isFull { return q>rear == MAX_SIZE 1;}
void enqueue { if qwe2 { printf; return; } if qwe2 { q>front = 0; } q>rear ; q>data = value;}
int dequeue { if qwe2 { printf; return 1; } int value = q>data; if { q>front = 1; q>rear = 1; } else { q>front ; } return value;}
int length { if qwe2 { return 0; } return q>rear q>front 1;}
void clearQueue { q>front = 1; q>rear = 1;}
void destroyQueue { free;}
int main { Queue q; initializeQueue;
enqueue; enqueue; enqueue;
printfqwe2; printfqwe2; printfqwe2;
clearQueue; printfqwe2;
destroyQueue;
return 0;}```
这个示例展示了如何创建一个队列,添加元素,检查队列长度,移除元素,清空队列,以及销毁队列。您可以根据自己的需求进行修改和扩展。
深入浅出C语言队列实现与应用
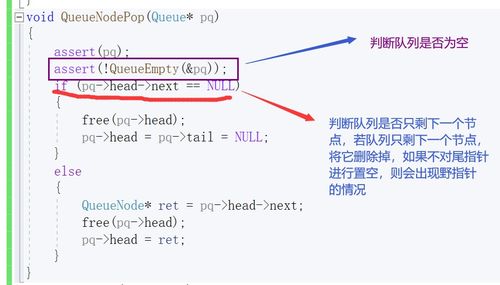
在计算机科学中,队列是一种重要的数据结构,它遵循“先进先出”(FIFO)的原则。本文将深入浅出地介绍C语言中队列的实现方法、基本操作以及在实际编程中的应用。
队列是一种线性表,它只允许在一端进行插入操作(称为队尾),在另一端进行删除操作(称为队头)。这种数据结构确保了元素按照插入的顺序被处理,即最先插入的元素将最先被处理。
在C语言中,队列可以通过数组来实现。以下是一个简单的队列结构体定义:
```c
typedef struct {
int array;
int front; // 队头指针
int rear; // 队尾指针
int size; // 队列最大容量
} Queue;
队列的初始化、入队和出队操作如下:
```c
// 初始化队列
void QueueInit(Queue q, int capacity) {
q->array = (int )malloc(capacity sizeof(int));
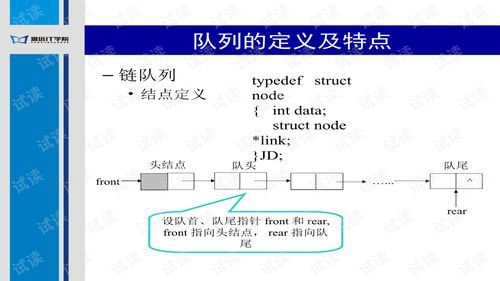
q->front = 0;
q->rear = -1;
q->size = capacity;
// 入队操作
void QueuePush(Queue q, int value) {
if ((q->rear 1) % q->size == q->front) {
// 队列已满
return;
}
q->rear = (q->rear 1) % q->size;
q->array[q->rear] = value;
// 出队操作
int QueuePop(Queue q) {
if (q->front == q->rear) {
// 队列为空
return -1;
}
int value = q->array[q->front];
q->front = (q->front 1) % q->size;
return value;
除了数组实现,队列也可以通过链表来实现。链表实现队列的优点是队列的大小不受限制,但缺点是插入和删除操作的时间复杂度为O(1)。
```c
typedef struct QueueNode {
int data;
struct QueueNode next;
} QueueNode;
typedef struct {
QueueNode front;
QueueNode rear;
} Queue;
// 初始化队列
void QueueInit(Queue q) {
q->front = NULL;
q->rear = NULL;
// 入队操作
void QueuePush(Queue q, int value) {
QueueNode newNode = (QueueNode )malloc(sizeof(QueueNode));
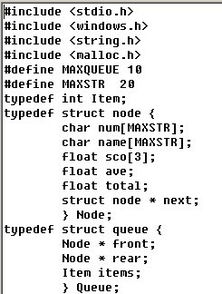
newNode->data = value;
newNode->next = NULL;
if (q->rear == NULL) {
q->front = newNode;
q->rear = newNode;
} else {
q->rear->next = newNode;
q->rear = newNode;
}
// 出队操作
int QueuePop(Queue q) {
if (q->front == NULL) {
// 队列为空
return -1;
}
QueueNode temp = q->front;
int value = temp->data;
q->front = q->front->next;
if (q->front == NULL) {
q->rear = NULL;
}
free(temp);
return value;
任务调度:在多线程编程中,队列可以用来存储待处理的任务,线程可以从队列中取出任务进行处理。
生产者-消费者模型:在多线程编程中,队列可以用来实现生产者和消费者之间的数据交换。
缓冲区管理:在数据传输过程中,队列可以用来存储临时数据,以实现数据的平滑传输。
队列是一种重要的数据结构,在C语言中可以通过数组或链表来实现。本文介绍了队列的基本概念、实现方法以及在实际编程中的应用。掌握队列的相关知识对于提高编程能力具有重要意义。