在React中,父组件可以通过使用`ref`来调用子组件的方法。这里是如何做到这一点的步骤:
1. 在父组件中创建一个`ref`。2. 将这个`ref`作为属性传递给子组件。3. 在子组件中,使用`ref`来获取父组件传递的属性。4. 在子组件中,使用`this.props.ref`来调用父组件的方法。
下面是一个简单的示例:
```jsximport React, { Component } from 'react';
class ParentComponent extends Component { constructor { super; this.childRef = React.createRef; }
callChildMethod = => { this.childRef.current.childMethod; }
render { return ; }}
class ChildComponent extends Component { childMethod = => { console.log; }
render { return Child Component; }}
export default ParentComponent;```
在这个示例中,`ParentComponent`有一个`ref`,它被传递给`ChildComponent`。当父组件的按钮被点击时,它会调用`callChildMethod`方法,这个方法又调用了子组件的`childMethod`方法。
React父组件调用子组件方法详解
在React开发中,组件间的通信是必不可少的。父组件与子组件之间的通信方式有很多种,其中一种常见的方式就是父组件调用子组件的方法。本文将详细介绍如何在React中实现父组件调用子组件的方法。
在React中,组件间通信主要有以下几种方式:
1. 父传子:通过props将数据传递给子组件。
2. 子传父:子组件通过调用父组件传递到子组件的方法向父组件传递消息。
3. 事件委托:利用事件冒泡机制,在父组件中处理子组件的事件。
本文将重点介绍第二种方式,即父组件调用子组件的方法。
二、父组件调用子组件方法的实现方式
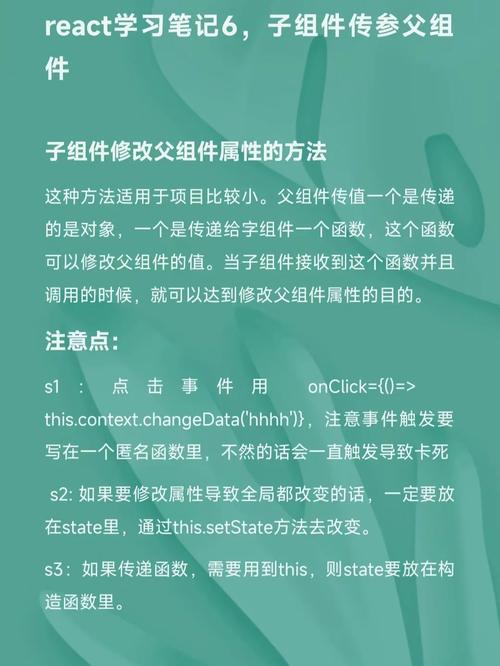
在React中,父组件调用子组件的方法主要有以下几种实现方式:
1. 使用ref
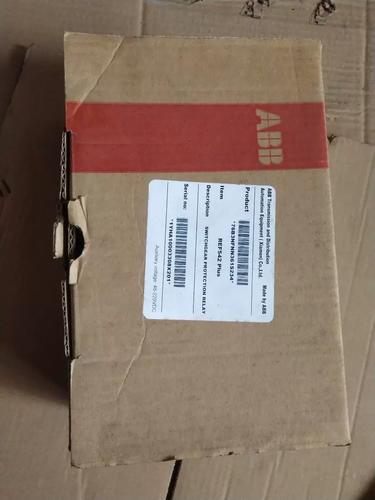
在React中,可以通过ref来访问子组件的DOM节点或实例。以下是一个使用ref调用子组件方法的示例:
```javascript
import React, { useRef } from 'react';
// 子组件
function ChildComponent() {
const childRef = useRef();
const callChildMethod = () => {
childRef.current.childMethod();
};
return (
调用子组件方法
);
// 父组件
function ParentComponent() {
return (
);
在上面的示例中,父组件通过ref属性将一个引用传递给子组件。子组件通过ref.current访问到父组件传递的引用,并调用其中的方法。
2. 使用回调函数
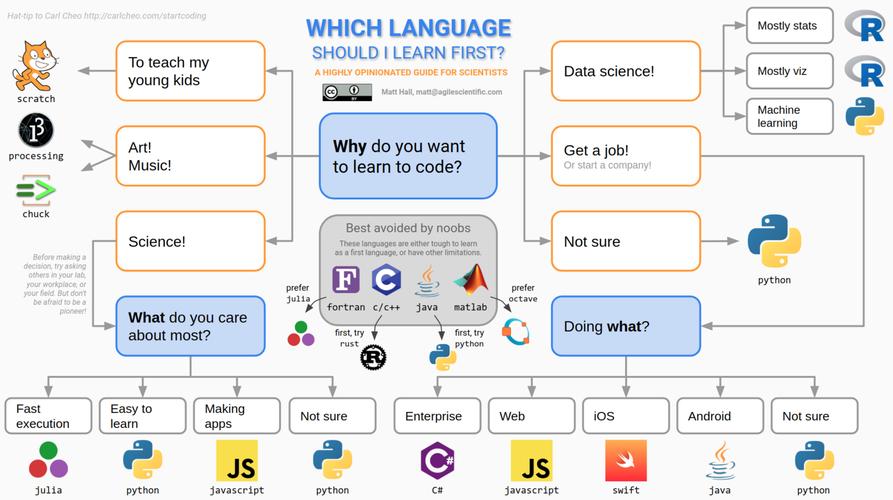
除了使用ref,还可以通过将回调函数传递给子组件,并在子组件中调用这个回调函数来实现父组件调用子组件的方法。
```javascript
import React, { useState } from 'react';
// 子组件
function ChildComponent(onCall) {
const callChildMethod = () => {
onCall();
};
return (
调用子组件方法
);
// 父组件
function ParentComponent() {
const [count, setCount] = useState(0);
const handleChildMethod = () => {
setCount(count 1);
};
return (
调用次数:{count}
);
在上面的示例中,父组件通过props将一个回调函数传递给子组件。子组件在需要的时候调用这个回调函数,从而实现父组件调用子组件的方法。
3. 使用自定义事件
除了以上两种方式,还可以通过自定义事件来实现父组件调用子组件的方法。
```javascript
import React, { useState } from 'react';
// 子组件
function ChildComponent() {
const callChildMethod = () => {
const event = new CustomEvent('childMethod', { detail: '子组件方法被调用' });
document.dispatchEvent(event);
};
return (
调用子组件方法
);
// 父组件
function ParentComponent() {
const handleChildMethod = (event) => {
console.log(event.detail);
};
return (
{`document.addEventListener('childMethod', handleChildMethod)`}
);
在上面的示例中,子组件通过自定义事件来通知父组件方法被调用。父组件通过监听这个自定义事件来处理子组件传递的消息。